Swift Animated Floating Menu
Add an Animated, Floating Menu to Any View in Your App

Scenario
Our app will allow users to sort the Books table view by utilising an animated, floating menu. The menu is triggered by tapping a ‘hamburger’ menu button, and offers 4 sort in-place criteria: By Title, Author, Series, and (User) Rating.
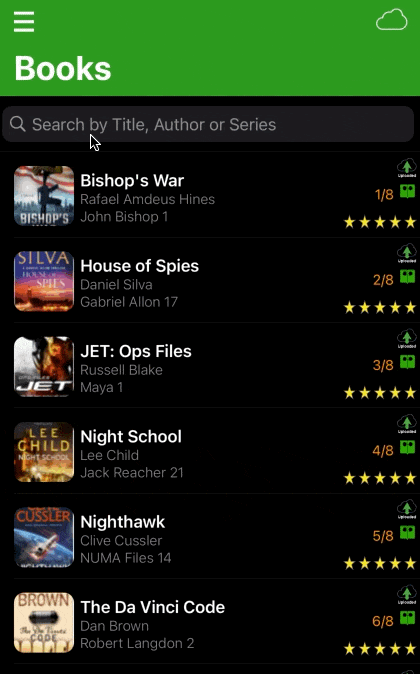
Technique
We design the menu in its own separate view, AnimatedMenuViewController.swift, then overlay it with a transparent background on top of the table view. The animation is provided by Meng To’s Spring package from GitHub. As the table sort happens ‘in place’, we refresh the table view in viewWillAppear().
The BooksTableViewController.swift acts as a delegate to AnimatedMenuViewController, so that any user actions on the menu can be detected by BooksTableViewController and the sort logic is executed.
Audience
The article is for Swift developers who seek complete, proven, code-centric solutions to speed up their development projects. The code snippets below can be used with minimal customisation.
App Model
We based the article on the AppsGym Books model app, published on Apple’s App Store (as 8Books), and you can download the complete Xcode project on AppsGym.com, for free.
User Interfaces
There are 2 views to consider: the Menu view (the top/overlay) and the Table view (the bottom/underlay). The Menu view will show the menu options clearly, with a transparent or translucent background, so that it does not cover the underlying Table view.
AnimatedMenuViewController.swift includes the menu itself as a DesignableView: SpringView (from Meng To’s Spring package on GitHub; meng@designcode.io), thus allowing the squeezeDown animation (image below). You may choose any other animation package or code your own, of course.

BooksTableViewController.swift displays our app Books (from Core Data) and shows the top left-hand icon of the Hamburger Menu, which triggers (via segue) the Floating Menu.

Logic
Firstly, we design the Floating Menu to perform 4 actions: Sort the underlying UITableView, in-place, by Book Title, Author, Series, or (User) Rating. We choose a square design, but you can place the buttons in any stack (horizontal, vertical, etc.).
Our AnimatedMenuViewController.swift class will have a delegate protocol, AnimatedMenuViewControllerDelegate, with 4 functions that correspond to the 4 menu actions.
We then construct @IBAction for each button touched/tapped, simply passing to the calling view (BooksTableViewController) an indication of which menu button has been touched/tapped. Then we dismiss the Floating Menu, so the UITableView, knowing which button has been touched/tapped, will execute the corresponding action on the displayed records.
Example: the user taps the (sort) By Author button, which the Menu delegate protocol will pass to the Books table view. The UITableView, being a delegate, will ‘sense’ the button tap and execute the relevant logic code (sort the displayed book records by author and refresh the view).
We also need a way to dismiss the menu if the user decides not to use it. So, we’ll add a transparent button behind the menu, invisible but covering the whole view, and connect it to a menu dismissal logic. Hence, if the user taps anywhere outside the menu ‘box’, the menu will be dismissed and no action taken. We can choose to animate the menu dismissal as well, rather than a sudden disappearance, and in our example we will make it ‘fall’ down the screen.
Code
Code: Animated Menu
The Swift code snippets below are extracts from AnimatedMenuViewController.swift, which shows the menu and sets up the delegate protocol functions.
AnimatedMenuViewController.swift
Code: Table View
BooksTableViewController.swift viewWillAppear Checks the selected user menu sort option, defines the Sort Descriptors for each menu option, retrieves the Core Data records, sorts them and refresh the table view by reloadData().
BooksTableViewController.swift viewWillAppear()
prepare(for segue..) : Checks if the segue identifier is ‘MenuSegue’ and launches the menu.
BooksTableViewController.swift prepare(for segue: ..)
BooksTableViewController.swift Delegate Functions
The 2 functions below are specific for Series and SeriesNo sorting in menuViewControllerDidTouchButton3.
The article covered the complete setup, logic and code to implement an animated, floating menu. The example we used was a sort-in-place of a displayed table view of books.

However, the floating menu can be used in/over any view of your app, animate and float from any direction, and execute any action, utilising the same principles of Delegates and Protocols. For example: in some apps, we utilised a floating menu as a Media Menu with options for Photo Album, Camera, Audio (Microphone), Video, and Share.
Hope you find the Floating Menu useful in your app. Thanks for reading!